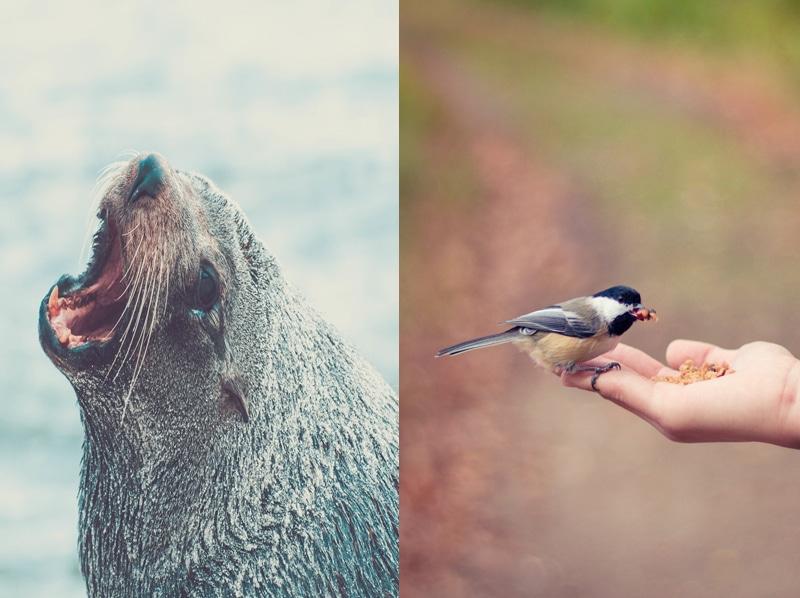
Reinventing Existing Products – Big Bite vs Small Nibble Rewrites
Big-bang rewrites (even with AI) just create tomorrows legacy code base faster
Read full articleBig-bang rewrites (even with AI) just create tomorrows legacy code base faster
Read full article[A Personal Retrospective on the Agile 2009
Read full articleEven if you haven't been laid off, I would start preparing now. You should start building
Read full articleStrong language like 'Rotten Apple' implies you're already convinced
Read full article- Does TDD really work? I've written about this before: [Advantages of
Read full articleGames that demonstrate process improvement, early delivery, small batches, and cross-functional benefits
Read full articleA few years ago [Bas Vodde](external:https://twitter.com/basvodde?lang=en) defined a simple test to
Read full articleA few weeks ago I ran my first Coding Dojo/Randori and
Read full articleWe ran our first TDD Randori session at lunch
Read full articleI keep on stumbling across posts about Agile 2008 and thought it might be worth sharing.
Read full articleI already wrote about my top sessions for InfoQ so I will just link to them instead of
Read full articleAt Agile 2007, I attended Jean Tabaka's (author of [Collaboration
Read full articleEffective coaching requires recognizing conflict levels, balancing skills/challenges
Read full articleLet's start by looking back to where this idea stems from. As best I can tell objections
Read full article- Why do you need this boolean named retVal? Could it be eliminated the use of early
Read full articleAs it stands today the [Catalog](external:https://scrumcommunity.pbwiki.com/Scrum+Smells) contains
Read full articleCollective Code Ownership isn't chaos, but team-based responsibility with shared standards
Read full articleDon't estimate task hours It doesn't work
Read full articleHowever in using a tool we miss the benefits of cards posted on a whiteboard/corkboard in
Read full articleCode Reviews mostly find problems that good tools could spot. Pairing changes that
Read full articleMeetings need simple ground rules and a clear agenda
Read full articleWorking at a distance is hard. There is a reason all the Agile methodologies recommend
Read full articleWhy Does Scrum work? Why do any of the Agile methodologies work? How does Scrum help teams
Read full articleExplore what Scrum is and how to make it work for you in our Scrum Certification training. Hands-on learning will guide you to improve teamwork, deliver quick feedback, and achieve better products and results.
Focuses on the role of the team and the ScrumMaster. Get the skills and practical experience necessary to improve teamwork, take the exam, and advance your career with a certification that is in high demand today. Often the best fit for anyone new to Scrum.
Learn on-the-job applications of key Scrum concepts, skills, principles, along with practical solutions that you can apply the next day for difficult, real-life situations.
Everything you need to earn your Scrum Alliance® ScrumMaster certification, including exam fee and membership, and so much more.
With focus on the challenges that real teams face, and tools to dig deeper. You don’t need more boring Scrum theory. You need something you can sink your teeth into to see immediate results.
This workshop is not just for software development or people with a computer science degree. We’ve helped many non-software teams with Scrum.
Use Scrum knowledge to standout at work, get paid more, and impress your customer, all without burning out.
Our active Scrum community forum is a safe place to ask questions. Long after you earn the Certified Scrum Master certification, you will have access to the forum, course materials, and additional valuable resources.